I'm trying to create a java program that logs me into neopets. but I'm running into some problems. is anyone here familiar enough with java to be of some help?
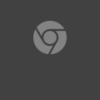
Can someone help me with java post requests?
#1
Posted 10 November 2013 - 11:11 PM
#2
Posted 10 November 2013 - 11:24 PM
Got any code so far?
If it's just a basic POST request I'd suggest checking out stackoverflow, as this has surely been asked before.
#3
Posted 10 November 2013 - 11:45 PM
Here is neopets login form:
<form method="post" action="/login.phtml"> <input type="hidden" id="templateLoginDest" name="destination" value="%2F"> <table style="width: 350px;" align="center"> <tr> <td valign="top" width="100px"><b>Username:</b></td> <td valign="top"><input type="text" name="username" id="templateLoginPopupUsername" size="30"></td> </tr> <tr> <td valign="top"><b>Password:</b></td> <td valign="top"><input type="password" name="password" size="30"><br><a href="/account/passwordreset.phtml" style="font-size: 8pt;">Forgot Password?</a><br><br></td> </tr> <tr> <td colspan="2" align="center"><input type="submit" value="Log In!"></td> </tr> </table> </form>
Edited by Nemecek, 10 November 2013 - 11:44 PM.
#4
Posted 11 November 2013 - 08:40 AM
Does HttpURLConnection handle cookies? If not you'll need to use a cookie manager otherwise you're always going to be logged out.
#5
Posted 11 November 2013 - 02:11 PM
I haven't looked into cookies yet. That's probably the way to do it but I'm wondering could I just resend my username and password each time I request a new page?
#6
Posted 12 November 2013 - 08:47 AM
I haven't looked into cookies yet. That's probably the way to do it but I'm wondering could I just resend my username and password each time I request a new page?
Nope. You might find some ideas on cookie handling in our old Java code snippets section:
http://www.neocodex....forum/137-java/
#7
Posted 12 November 2013 - 12:55 PM
Nope. You might find some ideas on cookie handling in our old Java code snippets section:
I didn't know we had a java forum. Thank you!
#8
Posted 12 November 2013 - 01:18 PM
I didn't know we had a java forum. Thank you!
It's really just an archive, it's not visible on the forum index so there's no point in posting in there.
#9
Posted 17 November 2013 - 09:16 PM
I feel as though I've made a lot of progress. But now again I'm stuck. Here's a portion of my http wrapper
#11
Posted 30 November 2013 - 12:29 AM
Here's another update:
I send my form data which looks something like this: destination=%252Findex.phtml&username=user&password=pass
I create and send headers with all the information Google Chrome shows me its sending (with the exception of the cookies)
-
Host:www.neopets.com
-
Origin:
-
Referer:
-
User-Agent:Mozilla/5.0 (Windows NT 6.2; WOW64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/31.0.1650.57 Safari/537.36
-
Accept:text/html,application/xhtml+xml,application/xml;q=0.9,image/webp,*/*;q=0.8
-
Accept-Encoding:gzip,deflate,sdch
-
Accept-Language:en-US,en;q=0.8
-
Cache-Control:max-age=0
-
Connection:keep-alive
-
Content-Length:69
-
Content-Type:application/x-www-form-urlencoded
-
Cookie: Cookies (This part I'm not sure about because although Google Chrome sends neopets a shit ton of cookies I don't send anything because it has nothing to do with my account it's just information regarding where I live and stuff)
Then my program outputs these as the Response headers:
-
Cache-Control:no-cache, must-revalidate
-
Connection:close
-
Content-Encoding:gzip
-
Content-Length:20
-
Content-Type:text/html; charset=UTF-8
-
Date:Sat, 30 Nov 2013 06:46:06 GMT
-
Expires:Mon, 26 Jul 1997 05:00:00 GMT
-
Last-Modified:Sat, 30 Nov 2013 06:46:06 GMT
-
Location:/index.phtml
-
p3p:
-
Pragma:no-cache
-
Server:Apache/2.2.9 (Unix) mod_ssl/2.2.9 OpenSSL/0.9.7a PHP/5.1.6
-
Set-Cookie:ssotoken=xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx; expires=Sun, 30-Nov-2014 06:46:06 GMT; path=/; domain=.neopets.com
-
Set-Cookie:neoremember=myusername; expires=Sun, 02-Mar-2014 20:59:26 GMT; path=/; domain=.neopets.com
-
Set-Cookie:wc_ids=0; expires=Thu, 29-Aug-2013 16:32:46 GMT; path=/; domain=.neopets.com
-
Set-Cookie:neologin=xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx; expires=Sun, 30-Nov-2014 06:46:06 GMT; path=/; domain=.neopets.com
-
Set-Cookie:toolbar=xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx; expires=Sun, 30-Nov-2014 06:46:06 GMT; path=/; domain=.neopets.com
-
Vary:Accept-Encoding,User-Agent
-
X-Powered-By:PHP/5.1.6
Edited by Nemecek, 30 November 2013 - 12:32 AM.
#12
Posted 30 November 2013 - 02:41 AM
Compare the headers you're sending side-by-side with the headers a browser sends when logging in and make sure they're substantially the same (especially the page where the data is being sent to and the data itself).
#13
Posted 30 November 2013 - 01:20 PM
Waser Lave Thank you for helping me out and being so quick to answer all the time.
However my headers are identical. The data being sent is identical and it is being sent to the right location. Other possible errors I thought of were:
-The order of the headers? Although I thought this didn't matter.
-The cookie header: My browser sends some cookies but my program only sends an empty string. Once the program logs in it stores the response cookies and would send that along with my future posts. I've tried copy pasting my cookies and sending that with my log in post but that failed as well.
-At the beginning of the post it says: "POST /login.phtml HTTP/1.1 " Do I have to do anything with the HTTP/1.1?
-The reason I believe I'm not logging in is because the page source code I get does not contain my username. Maybe I'm getting the wrong page source? I say this because when I put in my real name and password I get the neopets main page source code but when i type a invalid user and pass I get a bad password page.
I feel like I'm really close. I just need a little push!
#14
Posted 30 November 2013 - 01:23 PM
The order doesn't really matter too much, it shouldn't really matter if you send empty cookies to start with and you can ignore the HTTP/1.1 thing.
If it doesn't have your username what does the page source show? Try outputting the html and creating a html file and have a look if it's telling you anything.
#15
Posted 30 November 2013 - 02:07 PM
Okay so I used http://www.draac.com/htmltester.html to paste the html to and get a visual of the page:
This is what I get with a fake user:
http://postimg.org/image/5urthfiwn/
This is what I get with a fake pass:
http://postimg.org/image/43yeik4nb/
And this is what I get when I enter my real user and pass:
http://postimg.org/image/71xaspmmd/
It seems like i could maybe try just entering my username and then send a second post with my password. But I should be able to do it all in one post.
#16
Posted 30 November 2013 - 02:14 PM
You know you should be sending the request to login.phtml and not index.phtml, right?
#17
Posted 30 November 2013 - 02:37 PM
You know you should be sending the request to login.phtml and not index.phtml, right?
I use to have index.phtml in the referer but I got rid of it. The request gets sent to the url which you can see in my code is http://www.neopets.com/login.phtml
I must be sending it to the right place because when i do send false or incomplete information it tells me.
Edited by Nemecek, 30 November 2013 - 02:41 PM.
#18
Posted 30 November 2013 - 03:00 PM
Strange, if your headers are right, your data is right and your destination is right then I can't see how it wouldn't be working.
#19
Posted 30 November 2013 - 03:36 PM
Strange, if your headers are right, your data is right and your destination is right then I can't see how it wouldn't be working.
Thanks for the help.
#20
Posted 12 December 2013 - 06:47 AM
Okay I'm finally done exams, YAY!
I may have figured out what was wrong with my program. This is based purely on trial and error but it seems as though if i send my post request a second time it'll log me in. I think this happens because I believe that in order to log in you need to send the following cookies:
#21
Posted 12 December 2013 - 09:31 AM
Can you not just request another page after attempting to login and then checking if you're logged in on there?
#22
Posted 12 December 2013 - 09:52 AM
Can you not just request another page after attempting to login and then checking if you're logged in on there?
Thanks for the suggestion,
So I sent a GET request to go to the shopwizard and the html returned is fucked lol. I get returned a text looking something like this:
#23
Posted 12 December 2013 - 09:59 AM
Thanks for the suggestion,
So I sent a GET request to go to the shopwizard and the html returned is fucked
lol. I get returned a text looking something like this:
»?{ô?}?è[3üÓ[ÚùÎ^Óë@-q?^Wm¥ÿôºÊ+?¨×U^áI½®ê?îÔk¹ÿËõ©^?z©cõZ?çæ%޸йeNÕiBê?½P9?³t@?f©<Â6Ðíd¿ý#kg?Mò?{ÒØ´êдl¨²óôlW/è+?ÓÅEÛUk?ÓgþØsãÉIS?)?õÁNx-?x2/+?¾xo?(??g¿ûÛ¥¥0ÆÿS1KâáÒæF`×<?ãwÿ?»Ká??<=G×BîËÊ?ÎgÐ?¿ûÏ?·¬Ú±ÿó?ý?¿ÿÿòïÿÛßýþ¯ÿjY¹?Xî¿ÿåÿþÿju¹÷°^üý¿ùw¿ÿ?ÿø¿þöß.+tX§ÿï_ÿÍÿù¯÷ÿú¿d?ôúnÃοÍÜ¡ÆÚè}ÞÍÞ56ùºh'á)¨i?VXF]óá\hMTûç\¨'?aà¹C?c+åbµmmºÚí#[x»e?kçjÚê,ï0?E???Á1qÙ7P90QÉÎmu0?¸a|?2¡¬áNVí?ÀÚv&÷ÉrV`Kk5[ÒZ¿®ðA0#î?+)H?Î?¨:¿?PÌÕ|èûÀ?ÀRX8¡ã£ÕlÑ?~ |Ák[÷É ÏÀb·³?XA3buÄéé??ÿ_äL¾?ú¬?÷ÒÝIú8-??Åz²TÙÇ?«åé}f«?Úä)-bò?Ôéy ÏQÖÐO7ÿ(séGwú8ݪÃ? ? õ3*o?;}?q´¼?Ñ î?a0Û}æÏÐ?õÇêë?lù³zEÎlÒV¡?f'ÑQo?¿?9?£äê?:~K¯®?<oKÐBõÛõ×&Õûi'¡º?è+?2ý6ôfÃó¶¤?5oGå??·!ðSaÈGbìÆ°ä:?%ÁãÙ4?rÀUß µy?CÖå?òÒÏ?æbÑãµò 6Ê^â?T?,HnïaQCËØ9ئǫôöG?GLþ?Ã?§¸¾Tû¦KòT<I¢ª?ªÚÞ©åZ®ÙR¿µZÚÃ3Wê¹?k??6¾j?jté&Ì??U#?Î??-TÉÁH1?º??¹¾pì<9¾ò¸6¾Î*y´ØiÊ=þ'¯d]XüÔk®ïJ Ç>±a?ô]TÙÔXª? í¢?ë?§*ÉjÝlñ³ë2?ZtZ¿Rot±7{?³wuýëÔÛÅTª[ßM?³±ØerÀ©ó?í³È«®0èÆ·$£9Ó¤¿£ ¨3òÎÞ,?IÚ??ÎÍUZ¶ ?¥Ñ5Â?º ö??îó;Ãð¾5ö?ð®??ÖXÌý¸?1òGfEy%ñ8LÈ©G"?®VªGUDtþ/s(Ò½?P:nz|Þ¥?K(´ioñAx³?F=±X²%{N>Kfìuäk?'ÝxÕ)·%YßÞ¤«ß??â¥l4G?5?'ImÇ<N¡?ö?Êg¨?$Ò QËd»}$1¿«´Ì?Ï׿2þ¹TFúnûá¡??ú_í©ü?ÅÈnº©ÀÒ¥Ë:m?t?ÚMEâfQ?Ô©ÀV???SämÈKÝ®îÖ?I¯ü<rWÖ¥ïR*òî O&k±ºFK¶??r?¦?PÖ"£&¹j¯?ëÆ?4¡ÔXÅlQS??Rs?B??Ìa*A¹?9]xØISJyAnªsÁ´ãiǬ#×?§ÆAa\ü??[!-ª'ÜtD¶h?Ü2ý?ËÆþrIsxtøNÝ:¢£?í¤3kéùA>éé?-NÎéØ??h#$µVÔÌä?çn]OP9úÒX"¦Ù?u?°Û.vÜ^«Ð»Fß.8?ÜLÜTRÒ um¾?úmå?lA¢v??íwÊ£?ébOºá?Ü¡kxpU÷Ü+ù??E`)¾Ð6+å?2/?Zô¯?#95j$~"õ]s¶AQ}?Ë¥£(%?ßíì&¾âß;4ZüL³î?¾Ó?ù?J?Ø?J)?Z³®??k·eO\P?oTdûíý}*¡DA??ô??î(L.¡,z?Ë7äFÑ?3«??Ü@[2ȤI},ñQ?x`"§(£þÙ¿0?7??Xè._6¸r?úÌsG\»CQAyõ?ÍÝÖ?êÅ?¸1õO?â¬ÿÒ>¬gZ[¯¦??ðAϾ LrH?ßBWdäIÞË?]?¡KÈ¡?kªØ)С4&ër¶.ïÝy?çôB?~¨+?»Yx6é-B*?`©4%}?Õûl¢L?*a¨¹kå$dO?{?wypÑ4`ö:Ù??Ðr\,>F?ð?L.ñãt?"ä~Z@%ææzIdrÝH?HèêÃÀÅÊ6(¯££¼<Ⱦð??¢Sí?ûR5?nÒ[ÝØ¬ÀÅ4?öÆ1Ê? (?7A®1\¤O÷Ë?ðÛØ1û:ÜÆX`v$?f ?.VêÃÛ¹NÊ???C¼??&hóI87?}X>ÁktU&áWò2and it still says it's UTF-8
What headers did you send and receive? You should get a Content-Encoding header that will tell you what you need to do with this... Are you sending an Accept-Encoding header?
#24
Posted 12 December 2013 - 10:55 AM
What headers did you send and receive? You should get a Content-Encoding header that will tell you what you need to do with this... Are you sending an Accept-Encoding header?
Ah you nailed it!
I was using httpurlconnection.getContentType(); which returned to me UTF-8
but really I should have been using httpurlconnection.getEncodingContent(); which returns gzip and now it works
Thanks a lot I'm really excited that I've finally made progress.
Step 1: Login (check)
Step 2: Dominate Neopia ()
Step 3: Get Iced ()
Step 4: Start all over again ()
#25
Posted 12 December 2013 - 11:05 AM
This should fix your initial issue as well - if you decompress the gzip body you should then be able to perform a check on whether you're logged in or not.
Also, it's important that you have the right tooling when you're writing things like this.
Download Fiddler, it will listen to all of your web requests and responses and display them in an easy-to-read way, with the request and the response. Any requests your program makes will be listed when they are made.
You can also do some very cool things with it such as decompress third-party serialization techniques (AMF, for example), decrypt SSL, repeat certain requests, etc. You can save requests to look at later (which is very handy when you're writing a neopets program).
0 user(s) are reading this topic
0 members, 0 guests, 0 anonymous users